文件上传 - 比特日记
文件上传
上传
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
1import { common } from "@kit.AbilityKit"
2import fs from '@ohos.file.fs'
3import { BusinessError, request } from "@kit.BasicServicesKit"
4
5// 获取上下文
6let context = getContext(this) as common.UIAbilityContext
7// 缓存文件夹
8let cacheDir = context.cacheDir
9
10/**
11 * 封装文件上传
12 */
13export function FileUpload() {
14 // 准备需要上传的文件结构
15 let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
16 // 往文件中写入数据
17 fs.writeSync(file.fd, 'upload file test')
18 // 关闭写入流
19 fs.closeSync(file)
20
21 // 上传任务配置
22 let header = new Map<Object, string>()
23 // header.set('Content-type', 'multi/form-data')
24 // header.set('key2', 'value2')
25
26 // 上传文件对象
27 let files: Array<request.File> = [
28 // uri前缀internal://cache对应cacheDir目录
29 {
30 filename: 'test.txt',
31 name: 'file',
32 uri: 'internal://cache/test.txt',
33 type: 'file'
34 }
35 ]
36
37 // 文件上传时,传递参数
38 let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
39 let uploadConfig: request.UploadConfig = {
40 url: '',
41 header: header,
42 method: 'POST',
43 files: files,
44 data: data
45 }
46
47 // 将本地应用文件上传到网络服务器
48 try {
49 request.uploadFile(context, uploadConfig)
50 .then((uploadTask: request.UploadTask) => {
51 uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
52 for (let i = 0; i < taskStates.length; i++) {
53 console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
54 }
55 })
56 }, (err: BusinessError) => {
57 console.log(`upload file faile, ${err.name}-${err.message}`)
58 })
59 } catch (error) {
60 let err = error as BusinessError
61 console.log(`upload file faile, ${err.name}-${err.message}`)
62 }
63}
import { common } from "@kit.AbilityKit"
import fs from '@ohos.file.fs'
import { BusinessError, request } from "@kit.BasicServicesKit"
// 获取上下文
let context = getContext(this) as common.UIAbilityContext
// 缓存文件夹
let cacheDir = context.cacheDir
/**
* 封装文件上传
*/
export function FileUpload() {
// 准备需要上传的文件结构
let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
// 往文件中写入数据
fs.writeSync(file.fd, 'upload file test')
// 关闭写入流
fs.closeSync(file)
// 上传任务配置
let header = new Map<Object, string>()
// header.set('Content-type', 'multi/form-data')
// header.set('key2', 'value2')
// 上传文件对象
let files: Array<request.File> = [
// uri前缀internal://cache对应cacheDir目录
{
filename: 'test.txt',
name: 'file',
uri: 'internal://cache/test.txt',
type: 'file'
}
]
// 文件上传时,传递参数
let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
let uploadConfig: request.UploadConfig = {
url: '',
header: header,
method: 'POST',
files: files,
data: data
}
// 将本地应用文件上传到网络服务器
try {
request.uploadFile(context, uploadConfig)
.then((uploadTask: request.UploadTask) => {
uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
for (let i = 0; i < taskStates.length; i++) {
console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
}
})
}, (err: BusinessError) => {
console.log(`upload file faile, ${err.name}-${err.message}`)
})
} catch (error) {
let err = error as BusinessError
console.log(`upload file faile, ${err.name}-${err.message}`)
}
}
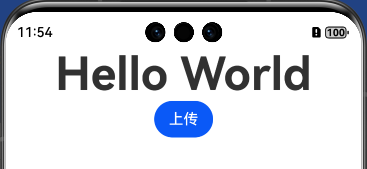
上传图片
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
1import { FileUpload, PickerPhoto } from '../utils/FileUpload';
2
3@Entry
4@Component
5struct Page07_Upload {
6 @State message: string = 'Hello World';
7 @State imgSrc: string | undefined = ''
8
9 build() {
10 Column() {
11 Text(this.message)
12 .id('Page07_UploadHelloWorld')
13 .fontSize($r('app.float.page_text_font_size'))
14 .fontWeight(FontWeight.Bold)
15 .alignRules({
16 center: { anchor: '__container__', align: VerticalAlign.Center },
17 middle: { anchor: '__container__', align: HorizontalAlign.Center }
18 })
19 .onClick(() => {
20 this.message = 'Welcome';
21 })
22
23 Button('上传').onClick(() => {
24 FileUpload()
25 })
26
27 Button('选择图片').onClick(async () => {
28 const imgUrl = await PickerPhoto()
29 this.imgSrc = imgUrl
30 })
31
32 Image(this.imgSrc)
33 .width(200)
34 .height(200)
35 }
36 .height('100%')
37 .width('100%')
38 }
39}
import { FileUpload, PickerPhoto } from '../utils/FileUpload';
@Entry
@Component
struct Page07_Upload {
@State message: string = 'Hello World';
@State imgSrc: string | undefined = ''
build() {
Column() {
Text(this.message)
.id('Page07_UploadHelloWorld')
.fontSize($r('app.float.page_text_font_size'))
.fontWeight(FontWeight.Bold)
.alignRules({
center: { anchor: '__container__', align: VerticalAlign.Center },
middle: { anchor: '__container__', align: HorizontalAlign.Center }
})
.onClick(() => {
this.message = 'Welcome';
})
Button('上传').onClick(() => {
FileUpload()
})
Button('选择图片').onClick(async () => {
const imgUrl = await PickerPhoto()
this.imgSrc = imgUrl
})
Image(this.imgSrc)
.width(200)
.height(200)
}
.height('100%')
.width('100%')
}
}
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
1import { common } from "@kit.AbilityKit"
2import fs from '@ohos.file.fs'
3import { BusinessError, request } from "@kit.BasicServicesKit"
4import { picker } from "@kit.CoreFileKit"
5
6// 获取上下文
7let context = getContext(this) as common.UIAbilityContext
8// 缓存文件夹
9let cacheDir = context.cacheDir
10
11/**
12 * 封装文件上传
13 */
14export function FileUpload() {
15 // 准备需要上传的文件结构
16 let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
17 // 往文件中写入数据
18 fs.writeSync(file.fd, 'upload file test')
19 // 关闭写入流
20 fs.closeSync(file)
21
22 // 上传任务配置
23 let header = new Map<Object, string>()
24 // header.set('Content-type', 'multi/form-data')
25 // header.set('key2', 'value2')
26
27 // 上传文件对象
28 let files: Array<request.File> = [
29 // uri前缀internal://cache对应cacheDir目录
30 {
31 filename: 'test.txt',
32 name: 'file',
33 uri: 'internal://cache/test.txt',
34 type: 'file'
35 }
36 ]
37
38 // 文件上传时,传递参数
39 let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
40 let uploadConfig: request.UploadConfig = {
41 url: '',
42 header: header,
43 method: 'POST',
44 files: files,
45 data: data
46 }
47
48 // 将本地应用文件上传到网络服务器
49 try {
50 request.uploadFile(context, uploadConfig)
51 .then((uploadTask: request.UploadTask) => {
52 uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
53 for (let i = 0; i < taskStates.length; i++) {
54 console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
55 }
56 })
57 }, (err: BusinessError) => {
58 console.log(`upload file faile, ${err.name}-${err.message}`)
59 })
60 } catch (error) {
61 let err = error as BusinessError
62 console.log(`upload file faile, ${err.name}-${err.message}`)
63 }
64}
65
66/**
67 * 从本地获取一张图片
68 */
69export async function PickerPhoto() {
70 // 打开系统图库
71 const options = new picker.PhotoSelectOptions
72 // 最多选中一张图片
73 options.maxSelectNumber = 1
74 // 限制用户只能访问相册
75 options.MIMEType = picker.PhotoViewMIMETypes.IMAGE_TYPE
76 // 获取选中图片地址
77 const pickerView = new picker.PhotoViewPicker
78 let urls = await pickerView.select(options)
79 if (urls.photoUris.length <= 0) {
80 return
81 }
82 let imgUrl = urls.photoUris[0]
83 console.log('imgUrl address:' + imgUrl)
84 return imgUrl
85}
import { common } from "@kit.AbilityKit"
import fs from '@ohos.file.fs'
import { BusinessError, request } from "@kit.BasicServicesKit"
import { picker } from "@kit.CoreFileKit"
// 获取上下文
let context = getContext(this) as common.UIAbilityContext
// 缓存文件夹
let cacheDir = context.cacheDir
/**
* 封装文件上传
*/
export function FileUpload() {
// 准备需要上传的文件结构
let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
// 往文件中写入数据
fs.writeSync(file.fd, 'upload file test')
// 关闭写入流
fs.closeSync(file)
// 上传任务配置
let header = new Map<Object, string>()
// header.set('Content-type', 'multi/form-data')
// header.set('key2', 'value2')
// 上传文件对象
let files: Array<request.File> = [
// uri前缀internal://cache对应cacheDir目录
{
filename: 'test.txt',
name: 'file',
uri: 'internal://cache/test.txt',
type: 'file'
}
]
// 文件上传时,传递参数
let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
let uploadConfig: request.UploadConfig = {
url: '',
header: header,
method: 'POST',
files: files,
data: data
}
// 将本地应用文件上传到网络服务器
try {
request.uploadFile(context, uploadConfig)
.then((uploadTask: request.UploadTask) => {
uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
for (let i = 0; i < taskStates.length; i++) {
console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
}
})
}, (err: BusinessError) => {
console.log(`upload file faile, ${err.name}-${err.message}`)
})
} catch (error) {
let err = error as BusinessError
console.log(`upload file faile, ${err.name}-${err.message}`)
}
}
/**
* 从本地获取一张图片
*/
export async function PickerPhoto() {
// 打开系统图库
const options = new picker.PhotoSelectOptions
// 最多选中一张图片
options.maxSelectNumber = 1
// 限制用户只能访问相册
options.MIMEType = picker.PhotoViewMIMETypes.IMAGE_TYPE
// 获取选中图片地址
const pickerView = new picker.PhotoViewPicker
let urls = await pickerView.select(options)
if (urls.photoUris.length <= 0) {
return
}
let imgUrl = urls.photoUris[0]
console.log('imgUrl address:' + imgUrl)
return imgUrl
}
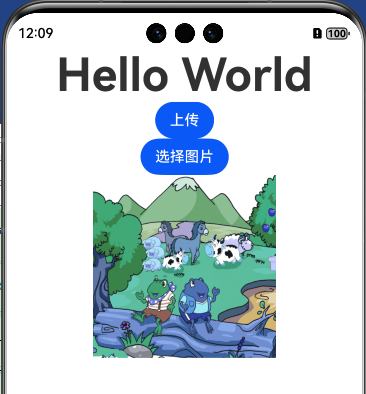
Copyright ©2010-2022 比特日记 All Rights Reserved.
Powered By 可尔物语
浙ICP备11005866号-12