图片上传 - 比特日记
图片上传
php
复制成功
1
2
3
1<?php
2 print_r($_FILES);
3?>
<?php
print_r($_FILES);
?>
选择图片
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
1import { FileUpload, FileUpload2, PickerPhoto } from '../utils/FileUpload';
2
3@Entry
4@Component
5struct Page07_Upload {
6 @State message: string = 'Hello World';
7 @State imgSrc: string | undefined = ''
8
9 build() {
10 Column() {
11 Text(this.message)
12 .id('Page07_UploadHelloWorld')
13 .fontSize($r('app.float.page_text_font_size'))
14 .fontWeight(FontWeight.Bold)
15 .alignRules({
16 center: { anchor: '__container__', align: VerticalAlign.Center },
17 middle: { anchor: '__container__', align: HorizontalAlign.Center }
18 })
19 .onClick(() => {
20 this.message = 'Welcome';
21 })
22
23 Button('上传').onClick(async () => {
24 await FileUpload2()
25 await FileUpload(this.imgSrc as string)
26 })
27
28 Button('选择图片').onClick(async () => {
29 const imgUrl = await PickerPhoto()
30 this.imgSrc = imgUrl
31 })
32
33 Image(this.imgSrc)
34 .width(200)
35 .height(200)
36 }
37 .height('100%')
38 .width('100%')
39 }
40}
import { FileUpload, FileUpload2, PickerPhoto } from '../utils/FileUpload';
@Entry
@Component
struct Page07_Upload {
@State message: string = 'Hello World';
@State imgSrc: string | undefined = ''
build() {
Column() {
Text(this.message)
.id('Page07_UploadHelloWorld')
.fontSize($r('app.float.page_text_font_size'))
.fontWeight(FontWeight.Bold)
.alignRules({
center: { anchor: '__container__', align: VerticalAlign.Center },
middle: { anchor: '__container__', align: HorizontalAlign.Center }
})
.onClick(() => {
this.message = 'Welcome';
})
Button('上传').onClick(async () => {
await FileUpload2()
await FileUpload(this.imgSrc as string)
})
Button('选择图片').onClick(async () => {
const imgUrl = await PickerPhoto()
this.imgSrc = imgUrl
})
Image(this.imgSrc)
.width(200)
.height(200)
}
.height('100%')
.width('100%')
}
}
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
1import { common } from "@kit.AbilityKit"
2import fs from '@ohos.file.fs'
3import { BusinessError, request } from "@kit.BasicServicesKit"
4import { picker } from "@kit.CoreFileKit"
5
6// 获取上下文
7let context = getContext(this) as common.UIAbilityContext
8// 缓存文件夹
9let cacheDir = context.cacheDir
10
11/**
12 * 上传图片
13 */
14export async function FileUpload(imgSrc: string) {
15 // 往文件中写入数据
16 const array = copyImgToCache(imgSrc)
17
18 // 上传任务配置
19 let header = new Map<Object, string>()
20 // header.set('Content-type', 'multi/form-data')
21 // header.set('key2', 'value2')
22
23 // 上传文件对象
24 let files: Array<request.File> = [
25 // uri前缀internal://cache对应cacheDir目录
26 {
27 filename: array[1],
28 name: 'file',
29 uri: array[0],
30 type: 'file'
31 }
32 ]
33
34 // 文件上传时,传递参数
35 let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
36 let uploadConfig: request.UploadConfig = {
37 url: 'http://www.yyxx.org/file.php',
38 header: header,
39 method: 'POST',
40 files: files,
41 data: data
42 }
43
44 // 将本地应用文件上传到网络服务器
45 try {
46 await request.uploadFile(context, uploadConfig)
47 .then((uploadTask: request.UploadTask) => {
48 uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
49 for (let i = 0; i < taskStates.length; i++) {
50 console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
51 }
52 })
53 }, (err: BusinessError) => {
54 console.log(`upload file faile, ${err.name}-${err.message}`)
55 })
56 } catch (error) {
57 let err = error as BusinessError
58 console.log(`upload file faile, ${err.name}-${err.message}`)
59 }
60}
61
62/**
63 * 封装文件上传
64 */
65export async function FileUpload2() {
66 // 准备需要上传的文件结构
67 let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
68 // 往文件中写入数据
69 fs.writeSync(file.fd, 'upload file test')
70 // 关闭写入流
71 fs.closeSync(file)
72
73 // 上传任务配置
74 let header = new Map<Object, string>()
75 // header.set('Content-type', 'multi/form-data')
76 // header.set('key2', 'value2')
77
78 // 上传文件对象
79 let files: Array<request.File> = [
80 // uri前缀internal://cache对应cacheDir目录
81 {
82 filename: 'test.txt',
83 name: 'file',
84 uri: 'internal://cache/test.txt',
85 type: 'file'
86 }
87 ]
88
89 // 文件上传时,传递参数
90 let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
91 let uploadConfig: request.UploadConfig = {
92 url: 'http://www.yyxx.org/file.php',
93 header: header,
94 method: 'POST',
95 files: files,
96 data: data
97 }
98
99 // 将本地应用文件上传到网络服务器
100 try {
101 await request.uploadFile(context, uploadConfig)
102 .then((uploadTask: request.UploadTask) => {
103 uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
104 for (let i = 0; i < taskStates.length; i++) {
105 console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
106 }
107 })
108 }, (err: BusinessError) => {
109 console.log(`upload file faile, ${err.name}-${err.message}`)
110 })
111 } catch (error) {
112 let err = error as BusinessError
113 console.log(`upload file faile, ${err.name}-${err.message}`)
114 }
115}
116
117/**
118 * 将文件拷贝到临时目录
119 */
120export function copyImgToCache(photoImgPath: string) {
121 const file = fs.openSync(photoImgPath, fs.OpenMode.READ_ONLY)
122 // 文件唯一标识
123 let fileFd = file.fd
124
125 let destPath = context.cacheDir
126 let fileName = Date.now().toString()
127 const ext = '.jpg'
128 let fullPath = cacheDir + '/' + fileName + ext
129 fs.copyFileSync(fileFd, fullPath)
130 return [`internal://cache/${fileName + ext}`, fileName + ext]
131}
132
133
134/**
135 * 从本地获取一张图片
136 */
137export async function PickerPhoto() {
138 // 打开系统图库
139 const options = new picker.PhotoSelectOptions
140 // 最多选中一张图片
141 options.maxSelectNumber = 1
142 // 限制用户只能访问相册
143 options.MIMEType = picker.PhotoViewMIMETypes.IMAGE_TYPE
144 // 获取选中图片地址
145 const pickerView = new picker.PhotoViewPicker
146 let urls = await pickerView.select(options)
147 if (urls.photoUris.length <= 0) {
148 return
149 }
150 let imgUrl = urls.photoUris[0]
151 console.log('imgUrl address:' + imgUrl)
152 return imgUrl
153}
import { common } from "@kit.AbilityKit"
import fs from '@ohos.file.fs'
import { BusinessError, request } from "@kit.BasicServicesKit"
import { picker } from "@kit.CoreFileKit"
// 获取上下文
let context = getContext(this) as common.UIAbilityContext
// 缓存文件夹
let cacheDir = context.cacheDir
/**
* 上传图片
*/
export async function FileUpload(imgSrc: string) {
// 往文件中写入数据
const array = copyImgToCache(imgSrc)
// 上传任务配置
let header = new Map<Object, string>()
// header.set('Content-type', 'multi/form-data')
// header.set('key2', 'value2')
// 上传文件对象
let files: Array<request.File> = [
// uri前缀internal://cache对应cacheDir目录
{
filename: array[1],
name: 'file',
uri: array[0],
type: 'file'
}
]
// 文件上传时,传递参数
let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
let uploadConfig: request.UploadConfig = {
url: 'http://www.yyxx.org/file.php',
header: header,
method: 'POST',
files: files,
data: data
}
// 将本地应用文件上传到网络服务器
try {
await request.uploadFile(context, uploadConfig)
.then((uploadTask: request.UploadTask) => {
uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
for (let i = 0; i < taskStates.length; i++) {
console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
}
})
}, (err: BusinessError) => {
console.log(`upload file faile, ${err.name}-${err.message}`)
})
} catch (error) {
let err = error as BusinessError
console.log(`upload file faile, ${err.name}-${err.message}`)
}
}
/**
* 封装文件上传
*/
export async function FileUpload2() {
// 准备需要上传的文件结构
let file = fs.openSync(cacheDir + '/test.txt', fs.OpenMode.READ_WRITE | fs.OpenMode.CREATE)
// 往文件中写入数据
fs.writeSync(file.fd, 'upload file test')
// 关闭写入流
fs.closeSync(file)
// 上传任务配置
let header = new Map<Object, string>()
// header.set('Content-type', 'multi/form-data')
// header.set('key2', 'value2')
// 上传文件对象
let files: Array<request.File> = [
// uri前缀internal://cache对应cacheDir目录
{
filename: 'test.txt',
name: 'file',
uri: 'internal://cache/test.txt',
type: 'file'
}
]
// 文件上传时,传递参数
let data: Array<request.RequestData> = [{ name: 'name', value: 'value' }]
let uploadConfig: request.UploadConfig = {
url: 'http://www.yyxx.org/file.php',
header: header,
method: 'POST',
files: files,
data: data
}
// 将本地应用文件上传到网络服务器
try {
await request.uploadFile(context, uploadConfig)
.then((uploadTask: request.UploadTask) => {
uploadTask.on('complete', (taskStates: Array<request.TaskState>) => {
for (let i = 0; i < taskStates.length; i++) {
console.log('upload complete taskState:' + JSON.stringify(taskStates[i]))
}
})
}, (err: BusinessError) => {
console.log(`upload file faile, ${err.name}-${err.message}`)
})
} catch (error) {
let err = error as BusinessError
console.log(`upload file faile, ${err.name}-${err.message}`)
}
}
/**
* 将文件拷贝到临时目录
*/
export function copyImgToCache(photoImgPath: string) {
const file = fs.openSync(photoImgPath, fs.OpenMode.READ_ONLY)
// 文件唯一标识
let fileFd = file.fd
let destPath = context.cacheDir
let fileName = Date.now().toString()
const ext = '.jpg'
let fullPath = cacheDir + '/' + fileName + ext
fs.copyFileSync(fileFd, fullPath)
return [`internal://cache/${fileName + ext}`, fileName + ext]
}
/**
* 从本地获取一张图片
*/
export async function PickerPhoto() {
// 打开系统图库
const options = new picker.PhotoSelectOptions
// 最多选中一张图片
options.maxSelectNumber = 1
// 限制用户只能访问相册
options.MIMEType = picker.PhotoViewMIMETypes.IMAGE_TYPE
// 获取选中图片地址
const pickerView = new picker.PhotoViewPicker
let urls = await pickerView.select(options)
if (urls.photoUris.length <= 0) {
return
}
let imgUrl = urls.photoUris[0]
console.log('imgUrl address:' + imgUrl)
return imgUrl
}
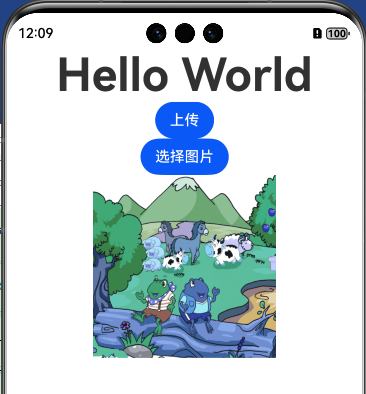
Copyright ©2010-2022 比特日记 All Rights Reserved.
Powered By 可尔物语
浙ICP备11005866号-12