懒加载 - 比特日记
懒加载
@LazyForEach
复制成功
1
2
3
4
5
6
7
1用户页面数据循环加载,实现数据懒加载、动态加载
2以屏幕可视区域为参考,配合滑动组件一起使用,只加载部分数据,节省性能
3内容滑出可视区域,销毁组件
4
5仅在List、Grid、Swiper、WaterFlow容器组件内使用
6cachedCount属性配置少量缓冲数据
7跟ForEach语法一样,数据约束不一样
用户页面数据循环加载,实现数据懒加载、动态加载
以屏幕可视区域为参考,配合滑动组件一起使用,只加载部分数据,节省性能
内容滑出可视区域,销毁组件
仅在List、Grid、Swiper、WaterFlow容器组件内使用
cachedCount属性配置少量缓冲数据
跟ForEach语法一样,数据约束不一样
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
1import { router } from '@kit.ArkUI';
2import { findDataByGetApi, findDataByPostApi } from '../apis/ProductApi';
3import { ParamsType } from '../utils/ParamsType';
4import { Evaluate, GoodDetail } from '../viewModel/GoodDetailModel';
5import { ProductDataModel } from '../viewModel/HomeModel';
6import { JSON } from '@kit.ArkTS';
7import { BreakPointConstants } from '../constants/BreakPointConstants';
8import { BreakPointSystem } from '../utils/BreakPointSystem';
9
10class BasicDataSource implements IDataSource {
11 // 存放监听器
12 private listener: DataChangeListener[] = []
13 // 存放数据
14 private originDataArray: string[] = []
15
16 // 计算数据量
17 public totalCount(): number {
18 return 0
19 }
20
21 // 获取数据
22 public getData(index: number): string {
23 return this.originDataArray[index];
24 }
25
26 // 增加listener
27 public registerDataChangeListener(listener: DataChangeListener): void {
28 if (this.listener.indexOf(listener) < 0) {
29 console.log('add listener')
30 this.listener.push(listener)
31 }
32 }
33
34 // 卸载listener
35 public unregisterDataChangeListener(listener: DataChangeListener): void {
36 const pos = this.listener.indexOf(listener)
37 if (pos >= 0) {
38 console.log('remove listener')
39 this.listener.splice(pos, 1)
40 }
41 }
42
43 // 重载所有子组件
44 notifyDataReload(): void {
45 this.listener.forEach(listener => {
46 listener.onDataReloaded()
47 })
48 }
49
50 notifyDataAdd(index: number): void {
51 this.listener.forEach(listener => {
52 listener.onDataChange(index)
53 })
54 }
55
56 notifyDataDelete(index: number): void {
57 this.listener.forEach(listener => {
58 listener.onDataDelete(index)
59 })
60 }
61
62 notifyDataMove(from: number, to: number): void {
63 this.listener.forEach(listener => {
64 listener.onDataMove(from, to)
65 })
66 }
67}
68
69class MyDataSource extends BasicDataSource {
70 private dataArray: string[] = []
71
72 public totalCount(): number {
73 return this.dataArray.length
74 }
75
76 // 获取数据
77 public getData(index: number): string {
78 return this.dataArray[index];
79 }
80
81 public addData(index: number, data: string): void {
82 this.dataArray.splice(index, 0, data)
83 this.notifyDataAdd(index)
84 }
85
86 public pushData(data: string): void {
87 this.dataArray.push(data)
88 this.notifyDataAdd(this.dataArray.length - 1)
89 }
90
91 public pushAllData(data: string[]): void {
92 for (let index = 0; index < data.length; index++) {
93 this.pushData(data[index])
94 }
95 }
96}
97
98@Entry({ routeName: 'detail' })
99@Component
100struct Page03_Detail {
101 private data: MyDataSource = new MyDataSource()
102
103 aboutToAppear(): void {
104 const array: string[] = []
105 for (let index = 0; index < 10000; index++) {
106 array.push(`Harmony ` + index)
107 }
108 this.data.pushAllData(array)
109 }
110
111 build() {
112 List({ space: 3 }) {
113 LazyForEach(this.data, (item: string) => {
114 ListItem() {
115 Row() {
116 Text(item).fontSize(50)
117 .onAppear(() => {
118 console.log('appear:' + item)
119 })
120 }
121 .margin({
122 left: 10,
123 right: 10
124 })
125 }
126 }, (item: string) => item)
127 }.cachedCount(3)
128 }
129}
import { router } from '@kit.ArkUI';
import { findDataByGetApi, findDataByPostApi } from '../apis/ProductApi';
import { ParamsType } from '../utils/ParamsType';
import { Evaluate, GoodDetail } from '../viewModel/GoodDetailModel';
import { ProductDataModel } from '../viewModel/HomeModel';
import { JSON } from '@kit.ArkTS';
import { BreakPointConstants } from '../constants/BreakPointConstants';
import { BreakPointSystem } from '../utils/BreakPointSystem';
class BasicDataSource implements IDataSource {
// 存放监听器
private listener: DataChangeListener[] = []
// 存放数据
private originDataArray: string[] = []
// 计算数据量
public totalCount(): number {
return 0
}
// 获取数据
public getData(index: number): string {
return this.originDataArray[index];
}
// 增加listener
public registerDataChangeListener(listener: DataChangeListener): void {
if (this.listener.indexOf(listener) < 0) {
console.log('add listener')
this.listener.push(listener)
}
}
// 卸载listener
public unregisterDataChangeListener(listener: DataChangeListener): void {
const pos = this.listener.indexOf(listener)
if (pos >= 0) {
console.log('remove listener')
this.listener.splice(pos, 1)
}
}
// 重载所有子组件
notifyDataReload(): void {
this.listener.forEach(listener => {
listener.onDataReloaded()
})
}
notifyDataAdd(index: number): void {
this.listener.forEach(listener => {
listener.onDataChange(index)
})
}
notifyDataDelete(index: number): void {
this.listener.forEach(listener => {
listener.onDataDelete(index)
})
}
notifyDataMove(from: number, to: number): void {
this.listener.forEach(listener => {
listener.onDataMove(from, to)
})
}
}
class MyDataSource extends BasicDataSource {
private dataArray: string[] = []
public totalCount(): number {
return this.dataArray.length
}
// 获取数据
public getData(index: number): string {
return this.dataArray[index];
}
public addData(index: number, data: string): void {
this.dataArray.splice(index, 0, data)
this.notifyDataAdd(index)
}
public pushData(data: string): void {
this.dataArray.push(data)
this.notifyDataAdd(this.dataArray.length - 1)
}
public pushAllData(data: string[]): void {
for (let index = 0; index < data.length; index++) {
this.pushData(data[index])
}
}
}
@Entry({ routeName: 'detail' })
@Component
struct Page03_Detail {
private data: MyDataSource = new MyDataSource()
aboutToAppear(): void {
const array: string[] = []
for (let index = 0; index < 10000; index++) {
array.push(`Harmony ` + index)
}
this.data.pushAllData(array)
}
build() {
List({ space: 3 }) {
LazyForEach(this.data, (item: string) => {
ListItem() {
Row() {
Text(item).fontSize(50)
.onAppear(() => {
console.log('appear:' + item)
})
}
.margin({
left: 10,
right: 10
})
}
}, (item: string) => item)
}.cachedCount(3)
}
}
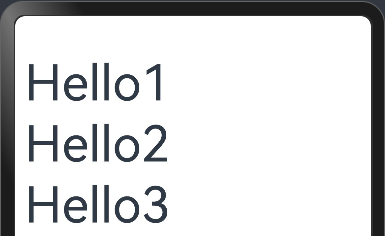
泛型
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
1export class BasicDataSource<T> implements IDataSource {
2 // 存放监听器
3 private listener: DataChangeListener[] = []
4 // 存放数据
5 private originDataArray: T[] = []
6
7 // 计算数据量
8 public totalCount(): number {
9 return 0
10 }
11
12 // 获取数据
13 public getData(index: number): T {
14 return this.originDataArray[index];
15 }
16
17 // 增加listener
18 public registerDataChangeListener(listener: DataChangeListener): void {
19 if (this.listener.indexOf(listener) < 0) {
20 console.log('add listener')
21 this.listener.push(listener)
22 }
23 }
24
25 // 卸载listener
26 public unregisterDataChangeListener(listener: DataChangeListener): void {
27 const pos = this.listener.indexOf(listener)
28 if (pos >= 0) {
29 console.log('remove listener')
30 this.listener.splice(pos, 1)
31 }
32 }
33
34 // 重载所有子组件
35 notifyDataReload(): void {
36 this.listener.forEach(listener => {
37 listener.onDataReloaded()
38 })
39 }
40
41 notifyDataAdd(index: number): void {
42 this.listener.forEach(listener => {
43 listener.onDataChange(index)
44 })
45 }
46
47 notifyDataDelete(index: number): void {
48 this.listener.forEach(listener => {
49 listener.onDataDelete(index)
50 })
51 }
52
53 notifyDataMove(from: number, to: number): void {
54 this.listener.forEach(listener => {
55 listener.onDataMove(from, to)
56 })
57 }
58}
export class BasicDataSource<T> implements IDataSource {
// 存放监听器
private listener: DataChangeListener[] = []
// 存放数据
private originDataArray: T[] = []
// 计算数据量
public totalCount(): number {
return 0
}
// 获取数据
public getData(index: number): T {
return this.originDataArray[index];
}
// 增加listener
public registerDataChangeListener(listener: DataChangeListener): void {
if (this.listener.indexOf(listener) < 0) {
console.log('add listener')
this.listener.push(listener)
}
}
// 卸载listener
public unregisterDataChangeListener(listener: DataChangeListener): void {
const pos = this.listener.indexOf(listener)
if (pos >= 0) {
console.log('remove listener')
this.listener.splice(pos, 1)
}
}
// 重载所有子组件
notifyDataReload(): void {
this.listener.forEach(listener => {
listener.onDataReloaded()
})
}
notifyDataAdd(index: number): void {
this.listener.forEach(listener => {
listener.onDataChange(index)
})
}
notifyDataDelete(index: number): void {
this.listener.forEach(listener => {
listener.onDataDelete(index)
})
}
notifyDataMove(from: number, to: number): void {
this.listener.forEach(listener => {
listener.onDataMove(from, to)
})
}
}
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
1import { BasicDataSource } from "./BasicDataSource";
2
3export class MyDataSource<T> extends BasicDataSource<T> {
4 private dataArray: T[] = []
5
6 public totalCount(): number {
7 return this.dataArray.length
8 }
9
10 // 获取数据
11 public getData(index: number): T {
12 return this.dataArray[index];
13 }
14
15 public addData(index: number, data: T): void {
16 this.dataArray.splice(index, 0, data)
17 this.notifyDataAdd(index)
18 }
19
20 public pushData(data: T): void {
21 this.dataArray.push(data)
22 this.notifyDataAdd(this.dataArray.length - 1)
23 }
24
25 public pushAllData(data: T[]): void {
26 for (let index = 0; index < data.length; index++) {
27 this.pushData(data[index])
28 }
29 }
30}
import { BasicDataSource } from "./BasicDataSource";
export class MyDataSource<T> extends BasicDataSource<T> {
private dataArray: T[] = []
public totalCount(): number {
return this.dataArray.length
}
// 获取数据
public getData(index: number): T {
return this.dataArray[index];
}
public addData(index: number, data: T): void {
this.dataArray.splice(index, 0, data)
this.notifyDataAdd(index)
}
public pushData(data: T): void {
this.dataArray.push(data)
this.notifyDataAdd(this.dataArray.length - 1)
}
public pushAllData(data: T[]): void {
for (let index = 0; index < data.length; index++) {
this.pushData(data[index])
}
}
}
对象
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
1import { MyDataSource } from '../utils/MyDataSource'
2import { ActivityDataModel } from '../viewModel/HomeModel'
3
4@Entry({ routeName: 'detail' })
5@Component
6struct Page05_LasyForEach {
7 private data: MyDataSource<ActivityDataModel> = new MyDataSource()
8 @State activityData: Array<ActivityDataModel> = [
9 {
10 id: '1',
11 name: '旅行',
12 image_src: 'app.media.startIcon',
13 navigator_url: '',
14 open_type: ''
15 }, {
16 id: '2',
17 name: '美食',
18 image_src: 'app.media.startIcon',
19 navigator_url: '',
20 open_type: ''
21 }, {
22 id: '3',
23 name: '周边',
24 image_src: 'app.media.startIcon',
25 navigator_url: '',
26 open_type: ''
27 }, {
28 id: '4',
29 name: '电子',
30 image_src: 'app.media.startIcon',
31 navigator_url: '',
32 open_type: ''
33 }, {
34 id: '5',
35 name: '话费',
36 image_src: 'app.media.startIcon',
37 navigator_url: '',
38 open_type: ''
39 }]
40
41 aboutToAppear(): void {
42 this.data.pushAllData(this.activityData)
43 }
44
45 build() {
46 List({ space: 3 }) {
47 LazyForEach(this.data, (item: ActivityDataModel) => {
48 ListItem() {
49 Row() {
50 Text(item.name).fontSize(50)
51 .onAppear(() => {
52 console.log('appear:' + item)
53 })
54 }
55 .margin({
56 left: 10,
57 right: 10
58 })
59 }
60 }, (item: ActivityDataModel) => item.id)
61 }.cachedCount(3)
62 }
63}
import { MyDataSource } from '../utils/MyDataSource'
import { ActivityDataModel } from '../viewModel/HomeModel'
@Entry({ routeName: 'detail' })
@Component
struct Page05_LasyForEach {
private data: MyDataSource<ActivityDataModel> = new MyDataSource()
@State activityData: Array<ActivityDataModel> = [
{
id: '1',
name: '旅行',
image_src: 'app.media.startIcon',
navigator_url: '',
open_type: ''
}, {
id: '2',
name: '美食',
image_src: 'app.media.startIcon',
navigator_url: '',
open_type: ''
}, {
id: '3',
name: '周边',
image_src: 'app.media.startIcon',
navigator_url: '',
open_type: ''
}, {
id: '4',
name: '电子',
image_src: 'app.media.startIcon',
navigator_url: '',
open_type: ''
}, {
id: '5',
name: '话费',
image_src: 'app.media.startIcon',
navigator_url: '',
open_type: ''
}]
aboutToAppear(): void {
this.data.pushAllData(this.activityData)
}
build() {
List({ space: 3 }) {
LazyForEach(this.data, (item: ActivityDataModel) => {
ListItem() {
Row() {
Text(item.name).fontSize(50)
.onAppear(() => {
console.log('appear:' + item)
})
}
.margin({
left: 10,
right: 10
})
}
}, (item: ActivityDataModel) => item.id)
}.cachedCount(3)
}
}
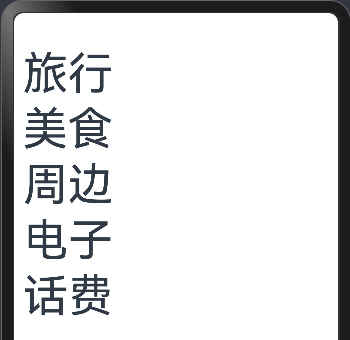
Copyright ©2010-2022 比特日记 All Rights Reserved.
Powered By 可尔物语
浙ICP备11005866号-12