引导 - 比特日记
引导
引导页
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
1import { BasicConstants } from '../constants/BasicConstants';
2import { router } from '@kit.ArkUI'
3import { BreakPointSystem } from '../utils/BreakPointSystem'
4import { BreakPointConstants } from '../constants/BreakPointConstants';
5
6@Entry
7@Component
8struct Page02_GuidePage {
9 @State message: string = 'Hello World';
10 @State count: number = 5
11 @StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = BreakPointConstants.BREAKPOINT_SM
12 private timer: number = 0
13 private breakPointSystem: BreakPointSystem = new BreakPointSystem()
14 @State flag: number = 1.2
15
16 aboutToAppear(): void {
17 this.breakPointSystem.register()
18 switch (this.currentBreakPoint) {
19 case BreakPointConstants.BREAKPOINT_SM:
20 this.flag *= 1
21 break
22 case BreakPointConstants.BREAKPOINT_MD:
23 this.flag *= 1.2
24 break
25 case BreakPointConstants.BREAKPOINT_LG:
26 this.flag *= 1.5
27 break
28 default:
29 this.flag *= 1
30 }
31 this.timer = setInterval(() => {
32 this.count--
33 if (this.count == 0) {
34 router.replaceUrl({
35 url: 'pages/Index'
36 })
37 }
38 }, 1000)
39 console.log(this.currentBreakPoint)
40 }
41
42 aboutToDisappear(): void {
43 this.breakPointSystem.unregister()
44 clearInterval(this.timer)
45 }
46
47 build() {
48 Stack({
49 alignContent: Alignment.TopEnd
50 }) {
51 Text(`倒计时:${this.count}`)
52 .margin({
53 right: 10
54 })
55 Flex({
56 direction: FlexDirection.Column,
57 alignItems: ItemAlign.Center
58 }) {
59 // logo
60 Column() {
61 Image($r('app.media.bird'))
62 .width(200 * this.flag)
63 .borderRadius(10)
64 }
65 .justifyContent(FlexAlign.Center)
66 .alignItems(HorizontalAlign.Center)
67 .flexGrow(1)
68
69 Image($r('app.media.startIcon'))
70 .width(28 * this.flag)
71 .objectFit(ImageFit.Contain)
72
73 Text('WoniuMall超市')
74 .fontColor('#66000000')
75 .fontSize(20 * this.flag)
76 .letterSpacing(16)
77 .margin({
78 top: 12,
79 bottom: 136
80 })
81 }
82 .width(BasicConstants.FULL_WIDTH)
83 .height(BasicConstants.FULL_HEIGHT)
84 }
85 }
86}
import { BasicConstants } from '../constants/BasicConstants';
import { router } from '@kit.ArkUI'
import { BreakPointSystem } from '../utils/BreakPointSystem'
import { BreakPointConstants } from '../constants/BreakPointConstants';
@Entry
@Component
struct Page02_GuidePage {
@State message: string = 'Hello World';
@State count: number = 5
@StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = BreakPointConstants.BREAKPOINT_SM
private timer: number = 0
private breakPointSystem: BreakPointSystem = new BreakPointSystem()
@State flag: number = 1.2
aboutToAppear(): void {
this.breakPointSystem.register()
switch (this.currentBreakPoint) {
case BreakPointConstants.BREAKPOINT_SM:
this.flag *= 1
break
case BreakPointConstants.BREAKPOINT_MD:
this.flag *= 1.2
break
case BreakPointConstants.BREAKPOINT_LG:
this.flag *= 1.5
break
default:
this.flag *= 1
}
this.timer = setInterval(() => {
this.count--
if (this.count == 0) {
router.replaceUrl({
url: 'pages/Index'
})
}
}, 1000)
console.log(this.currentBreakPoint)
}
aboutToDisappear(): void {
this.breakPointSystem.unregister()
clearInterval(this.timer)
}
build() {
Stack({
alignContent: Alignment.TopEnd
}) {
Text(`倒计时:${this.count}`)
.margin({
right: 10
})
Flex({
direction: FlexDirection.Column,
alignItems: ItemAlign.Center
}) {
// logo
Column() {
Image($r('app.media.bird'))
.width(200 * this.flag)
.borderRadius(10)
}
.justifyContent(FlexAlign.Center)
.alignItems(HorizontalAlign.Center)
.flexGrow(1)
Image($r('app.media.startIcon'))
.width(28 * this.flag)
.objectFit(ImageFit.Contain)
Text('WoniuMall超市')
.fontColor('#66000000')
.fontSize(20 * this.flag)
.letterSpacing(16)
.margin({
top: 12,
bottom: 136
})
}
.width(BasicConstants.FULL_WIDTH)
.height(BasicConstants.FULL_HEIGHT)
}
}
}
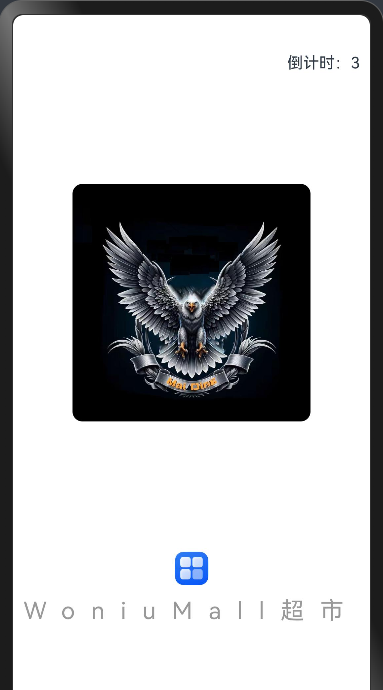
底部导航
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
1import { BasicConstants } from '../constants/BasicConstants'
2import { BreakPointSystem } from '../utils/BreakPointSystem'
3import { BreakPointConstants } from '../constants/BreakPointConstants'
4
5@Entry
6@Component
7struct Index {
8 @State message: string = 'Hello World';
9 private breakPointSystem = new BreakPointSystem()
10 @StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
11
12 aboutToAppear(): void {
13 this.breakPointSystem.register()
14 }
15
16 aboutToDisappear(): void {
17 this.breakPointSystem.unregister()
18 }
19
20 build() {
21 Column() {
22 Tabs({
23 barPosition: BarPosition.End
24 }) {
25 TabContent() {
26 Text('首页')
27 .fontSize(30)
28 }
29 .tabBar('首页')
30
31 TabContent() {
32 Text('推荐')
33 .fontSize(30)
34 }
35 .tabBar('推荐')
36
37 TabContent() {
38 Text('发现')
39 .fontSize(30)
40 }
41 .tabBar('发现')
42
43 TabContent() {
44 Text('我的')
45 .fontSize(30)
46 }
47 .tabBar('我的')
48 }
49 }
50 .height(BasicConstants.FULL_HEIGHT)
51 .width(BasicConstants.FULL_WIDTH)
52 }
53}
import { BasicConstants } from '../constants/BasicConstants'
import { BreakPointSystem } from '../utils/BreakPointSystem'
import { BreakPointConstants } from '../constants/BreakPointConstants'
@Entry
@Component
struct Index {
@State message: string = 'Hello World';
private breakPointSystem = new BreakPointSystem()
@StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
aboutToAppear(): void {
this.breakPointSystem.register()
}
aboutToDisappear(): void {
this.breakPointSystem.unregister()
}
build() {
Column() {
Tabs({
barPosition: BarPosition.End
}) {
TabContent() {
Text('首页')
.fontSize(30)
}
.tabBar('首页')
TabContent() {
Text('推荐')
.fontSize(30)
}
.tabBar('推荐')
TabContent() {
Text('发现')
.fontSize(30)
}
.tabBar('发现')
TabContent() {
Text('我的')
.fontSize(30)
}
.tabBar('我的')
}
}
.height(BasicConstants.FULL_HEIGHT)
.width(BasicConstants.FULL_WIDTH)
}
}
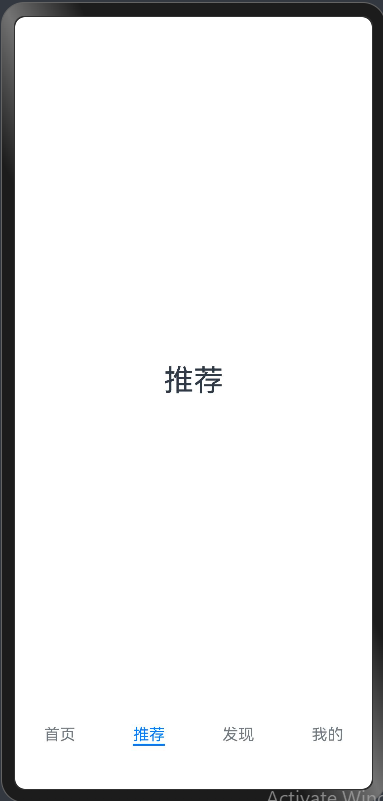
侧边导航
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
1import { BasicConstants } from '../constants/BasicConstants'
2import { BreakPointSystem } from '../utils/BreakPointSystem'
3import { BreakPointConstants } from '../constants/BreakPointConstants'
4
5@Entry
6@Component
7struct Index {
8 @State message: string = 'Hello World';
9 private breakPointSystem = new BreakPointSystem()
10 @StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
11
12 aboutToAppear(): void {
13 this.breakPointSystem.register()
14 }
15
16 aboutToDisappear(): void {
17 this.breakPointSystem.unregister()
18 }
19
20 build() {
21 Column() {
22 Tabs({
23 barPosition: BarPosition.Start
24 }) {
25 TabContent() {
26 Text('首页')
27 .fontSize(30)
28 }
29 .tabBar('首页')
30
31 TabContent() {
32 Text('推荐')
33 .fontSize(30)
34 }
35 .tabBar('推荐')
36
37 TabContent() {
38 Text('发现')
39 .fontSize(30)
40 }
41 .tabBar('发现')
42
43 TabContent() {
44 Text('我的')
45 .fontSize(30)
46 }
47 .tabBar('我的')
48 }
49 .vertical(true)
50 .barWidth(100)
51 .barHeight(200)
52 }
53 .height(BasicConstants.FULL_HEIGHT)
54 .width(BasicConstants.FULL_WIDTH)
55 }
56}
import { BasicConstants } from '../constants/BasicConstants'
import { BreakPointSystem } from '../utils/BreakPointSystem'
import { BreakPointConstants } from '../constants/BreakPointConstants'
@Entry
@Component
struct Index {
@State message: string = 'Hello World';
private breakPointSystem = new BreakPointSystem()
@StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
aboutToAppear(): void {
this.breakPointSystem.register()
}
aboutToDisappear(): void {
this.breakPointSystem.unregister()
}
build() {
Column() {
Tabs({
barPosition: BarPosition.Start
}) {
TabContent() {
Text('首页')
.fontSize(30)
}
.tabBar('首页')
TabContent() {
Text('推荐')
.fontSize(30)
}
.tabBar('推荐')
TabContent() {
Text('发现')
.fontSize(30)
}
.tabBar('发现')
TabContent() {
Text('我的')
.fontSize(30)
}
.tabBar('我的')
}
.vertical(true)
.barWidth(100)
.barHeight(200)
}
.height(BasicConstants.FULL_HEIGHT)
.width(BasicConstants.FULL_WIDTH)
}
}
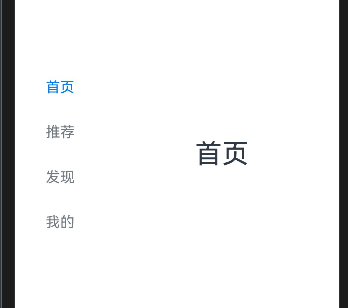
@Builder
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
1import { BasicConstants } from '../constants/BasicConstants'
2import { BreakPointSystem } from '../utils/BreakPointSystem'
3import { BreakPointConstants } from '../constants/BreakPointConstants'
4
5@Entry
6@Component
7struct Index {
8 @State message: string = 'Hello World';
9 @State currentIndex: number = 0
10 private breakPointSystem = new BreakPointSystem()
11 @StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
12
13 aboutToAppear(): void {
14 this.breakPointSystem.register()
15 }
16
17 aboutToDisappear(): void {
18 this.breakPointSystem.unregister()
19 }
20
21 // 函数内容为布局代码
22 @Builder
23 customBar(
24 title: string,
25 targetIndex: number,
26 selectImg: Resource,
27 normalImg: Resource
28 ) {
29 Column({
30 space: 3
31 }) {
32 Image(this.currentIndex == targetIndex ? selectImg : normalImg)
33 .size({
34 width: 28
35 })
36 Text(title)
37 .fontColor(this.currentIndex == targetIndex ? Color.Brown : Color.Pink)
38 }
39 .width('100%')
40 .height(50)
41 .justifyContent(FlexAlign.Center)
42 }
43
44 build() {
45 Column() {
46 Tabs({
47 barPosition: BarPosition.End
48 }) {
49 TabContent() {
50 Text('首页')
51 .fontSize(30)
52 }
53 .tabBar(this.customBar('首页', 0, $r('app.media.startIcon'), $r('app.media.startIcon')))
54
55 TabContent() {
56 Text('产品')
57 .fontSize(30)
58 }
59 .tabBar(this.customBar('产品', 1, $r('app.media.startIcon'), $r('app.media.startIcon')))
60
61 TabContent() {
62 Text('购物车')
63 .fontSize(30)
64 }
65 .tabBar(this.customBar('购物车', 2, $r('app.media.startIcon'), $r('app.media.startIcon')))
66
67 TabContent() {
68 Text('我的')
69 .fontSize(30)
70 }
71 .tabBar(this.customBar('我的', 3, $r('app.media.startIcon'), $r('app.media.startIcon')))
72 }
73 }
74 .height(BasicConstants.FULL_HEIGHT)
75 .width(BasicConstants.FULL_WIDTH)
76 }
77}
import { BasicConstants } from '../constants/BasicConstants'
import { BreakPointSystem } from '../utils/BreakPointSystem'
import { BreakPointConstants } from '../constants/BreakPointConstants'
@Entry
@Component
struct Index {
@State message: string = 'Hello World';
@State currentIndex: number = 0
private breakPointSystem = new BreakPointSystem()
@StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
aboutToAppear(): void {
this.breakPointSystem.register()
}
aboutToDisappear(): void {
this.breakPointSystem.unregister()
}
// 函数内容为布局代码
@Builder
customBar(
title: string,
targetIndex: number,
selectImg: Resource,
normalImg: Resource
) {
Column({
space: 3
}) {
Image(this.currentIndex == targetIndex ? selectImg : normalImg)
.size({
width: 28
})
Text(title)
.fontColor(this.currentIndex == targetIndex ? Color.Brown : Color.Pink)
}
.width('100%')
.height(50)
.justifyContent(FlexAlign.Center)
}
build() {
Column() {
Tabs({
barPosition: BarPosition.End
}) {
TabContent() {
Text('首页')
.fontSize(30)
}
.tabBar(this.customBar('首页', 0, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('产品')
.fontSize(30)
}
.tabBar(this.customBar('产品', 1, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('购物车')
.fontSize(30)
}
.tabBar(this.customBar('购物车', 2, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('我的')
.fontSize(30)
}
.tabBar(this.customBar('我的', 3, $r('app.media.startIcon'), $r('app.media.startIcon')))
}
}
.height(BasicConstants.FULL_HEIGHT)
.width(BasicConstants.FULL_WIDTH)
}
}
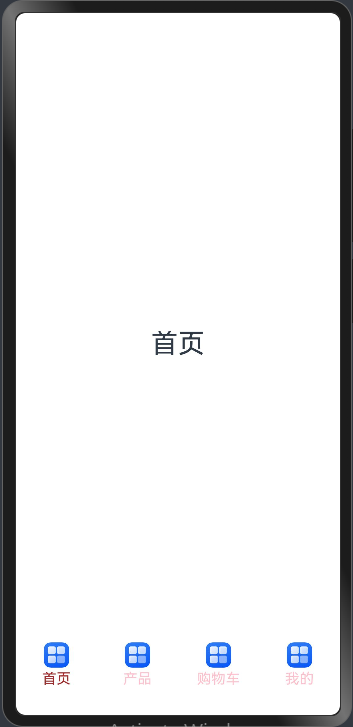
滑动
复制成功
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
1import { BasicConstants } from '../constants/BasicConstants'
2import { BreakPointSystem } from '../utils/BreakPointSystem'
3import { BreakPointConstants } from '../constants/BreakPointConstants'
4
5@Entry
6@Component
7struct Index {
8 @State message: string = 'Hello World';
9 @State currentIndex: number = 0
10 private tabController = new TabsController()
11 private breakPointSystem = new BreakPointSystem()
12 @StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
13
14 aboutToAppear(): void {
15 this.breakPointSystem.register()
16 }
17
18 aboutToDisappear(): void {
19 this.breakPointSystem.unregister()
20 }
21
22 // 函数内容为布局代码
23 @Builder
24 customBar(
25 title: string,
26 targetIndex: number,
27 selectImg: Resource,
28 normalImg: Resource
29 ) {
30 Column({
31 space: 3
32 }) {
33 Image(this.currentIndex == targetIndex ? selectImg : normalImg)
34 .size({
35 width: 28
36 })
37 Text(title)
38 .fontColor(this.currentIndex == targetIndex ? Color.Brown : Color.Pink)
39 }
40 .width('100%')
41 .height(50)
42 .justifyContent(FlexAlign.Center)
43 .onClick(() => {
44 this.currentIndex = targetIndex
45 // 控制器下标更新
46 this.tabController.changeIndex(targetIndex)
47 })
48 }
49
50 build() {
51 Column() {
52 Tabs({
53 barPosition: BarPosition.End,
54 controller: this.tabController
55 }) {
56 TabContent() {
57 Text('首页')
58 .fontSize(30)
59 }
60 .tabBar(this.customBar('首页', 0, $r('app.media.startIcon'), $r('app.media.startIcon')))
61
62 TabContent() {
63 Text('产品')
64 .fontSize(30)
65 }
66 .tabBar(this.customBar('产品', 1, $r('app.media.startIcon'), $r('app.media.startIcon')))
67
68 TabContent() {
69 Text('购物车')
70 .fontSize(30)
71 }
72 .tabBar(this.customBar('购物车', 2, $r('app.media.startIcon'), $r('app.media.startIcon')))
73
74 TabContent() {
75 Text('我的')
76 .fontSize(30)
77 }
78 .tabBar(this.customBar('我的', 3, $r('app.media.startIcon'), $r('app.media.startIcon')))
79 }
80 // 滑动界面触发更新
81 .onChange((index: number) => {
82 this.currentIndex = index
83 })
84 }
85 .height(BasicConstants.FULL_HEIGHT)
86 .width(BasicConstants.FULL_WIDTH)
87 .backgroundColor('#f1f3f5')
88 }
89}
import { BasicConstants } from '../constants/BasicConstants'
import { BreakPointSystem } from '../utils/BreakPointSystem'
import { BreakPointConstants } from '../constants/BreakPointConstants'
@Entry
@Component
struct Index {
@State message: string = 'Hello World';
@State currentIndex: number = 0
private tabController = new TabsController()
private breakPointSystem = new BreakPointSystem()
@StorageProp(BreakPointConstants.CURRENT_BREAKPOINT) currentBreakPoint: string = 'sm'
aboutToAppear(): void {
this.breakPointSystem.register()
}
aboutToDisappear(): void {
this.breakPointSystem.unregister()
}
// 函数内容为布局代码
@Builder
customBar(
title: string,
targetIndex: number,
selectImg: Resource,
normalImg: Resource
) {
Column({
space: 3
}) {
Image(this.currentIndex == targetIndex ? selectImg : normalImg)
.size({
width: 28
})
Text(title)
.fontColor(this.currentIndex == targetIndex ? Color.Brown : Color.Pink)
}
.width('100%')
.height(50)
.justifyContent(FlexAlign.Center)
.onClick(() => {
this.currentIndex = targetIndex
// 控制器下标更新
this.tabController.changeIndex(targetIndex)
})
}
build() {
Column() {
Tabs({
barPosition: BarPosition.End,
controller: this.tabController
}) {
TabContent() {
Text('首页')
.fontSize(30)
}
.tabBar(this.customBar('首页', 0, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('产品')
.fontSize(30)
}
.tabBar(this.customBar('产品', 1, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('购物车')
.fontSize(30)
}
.tabBar(this.customBar('购物车', 2, $r('app.media.startIcon'), $r('app.media.startIcon')))
TabContent() {
Text('我的')
.fontSize(30)
}
.tabBar(this.customBar('我的', 3, $r('app.media.startIcon'), $r('app.media.startIcon')))
}
// 滑动界面触发更新
.onChange((index: number) => {
this.currentIndex = index
})
}
.height(BasicConstants.FULL_HEIGHT)
.width(BasicConstants.FULL_WIDTH)
.backgroundColor('#f1f3f5')
}
}
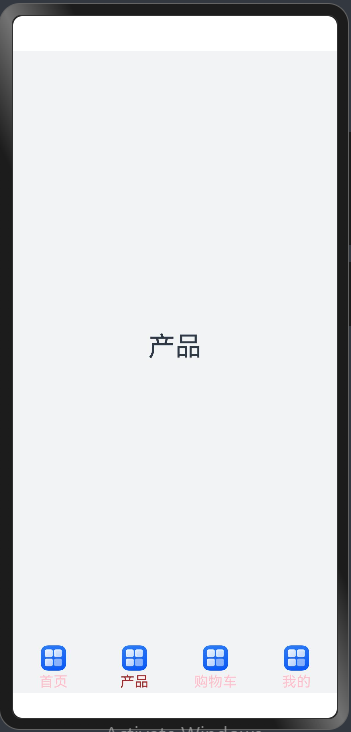
Copyright ©2010-2022 比特日记 All Rights Reserved.
Powered By 可尔物语
浙ICP备11005866号-12